5. Explanation of the UDP-Client Example#
1. Environment Preparation#
To ensure a smooth demonstration of UDP communication, we need to confirm that the following environment is correctly configured:
1.1 Hardware Connection#
Ensure that your CanMV development board and computer are connected to the same router or switch via Ethernet cable to form a local area network (LAN).
Ensure that the router or switch is working properly to guarantee network stability.
1.2 Disable Firewall#
To avoid the firewall blocking UDP communication, it is recommended to temporarily disable the computer’s firewall.
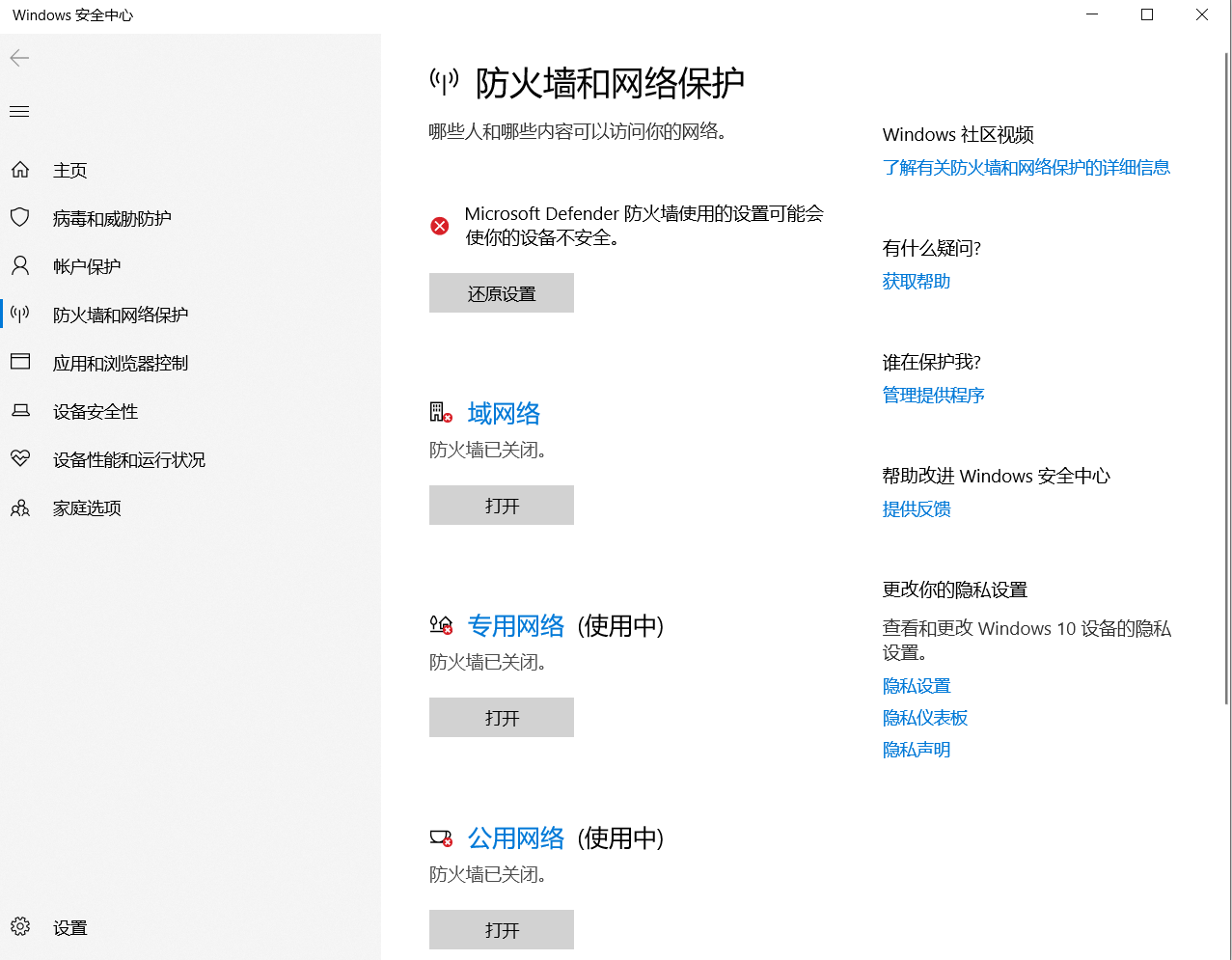
1.3 Tools Preparation#
Download and install NetAssist Network Debugging Assistant as a network communication testing tool to help with sending and receiving network data.
1.4 Obtain IP Address#
Open the command prompt (CMD), enter
ipconfig
, and record the IP address of the computer’s network card for subsequent configuration and testing.
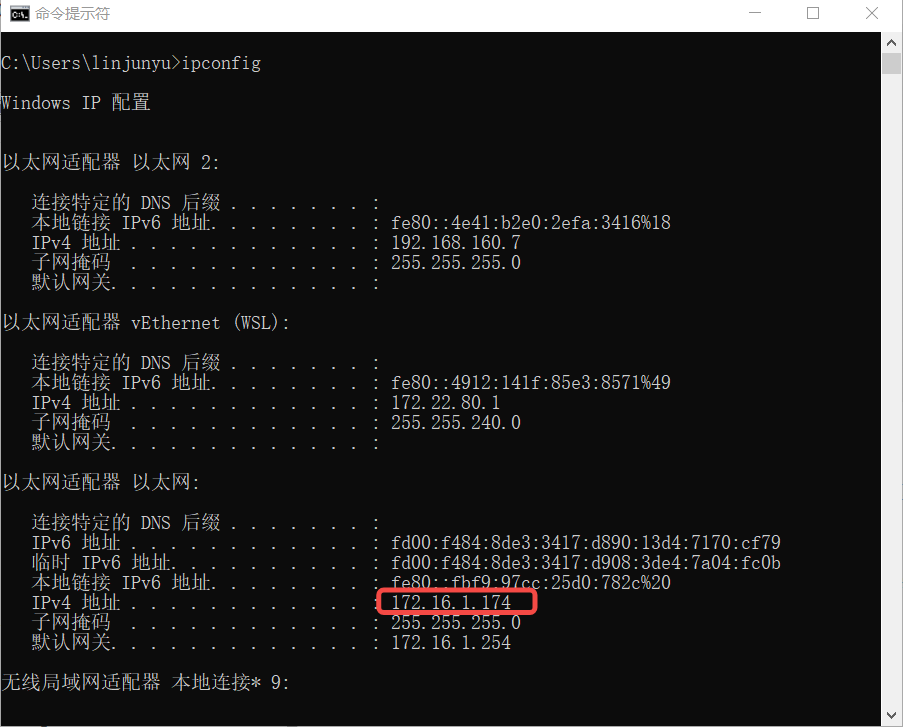
2. Client Example Analysis#
This UDP client example demonstrates how to create a simple UDP client, including connecting to a server, sending data, and closing the connection. You can learn the basic methods of building UDP communication applications through this example.
2.1 Import Necessary Libraries#
import socket
import network
import time
The
socket
library is responsible for creating network communication sockets.The
network
library is used to configure network interfaces, such as enabling LAN or WLAN.The
time
library provides delay operations, usually used to control data sending frequency or timeout handling.
2.2 Configure Network Interface#
def network_use_wlan(is_wlan=True):
if is_wlan:
sta = network.WLAN(0)
sta.connect("Canaan", "Canaan314")
print(sta.status())
while sta.ifconfig()[0] == '0.0.0.0':
os.exitpoint()
print(sta.ifconfig())
ip = sta.ifconfig()[0]
return ip
else:
a = network.LAN()
if not a.active():
raise RuntimeError("LAN interface is not active.")
a.ifconfig("dhcp")
print(a.ifconfig())
ip = a.ifconfig()[0]
return ip
This function configures the network interface based on whether a wireless network (WLAN) or a wired network (LAN) is chosen, with the following steps:
WLAN Mode: Attempts to connect to a Wi-Fi network and waits to obtain a valid IP address before returning.
LAN Mode: Activates the LAN interface and uses DHCP mode to obtain an IP address.
2.3 Create UDP Socket#
# Get the server's IP and port number
ai = socket.getaddrinfo('172.16.1.174', 8080)
print("Address infos:", ai)
addr = ai[0][-1] # Extract IP and port number
print("Connecting to address:", addr)
# Create UDP socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
Here, the server’s IP and port information is obtained through socket.getaddrinfo
, and the address and port number are extracted. Then, a UDP socket is created.
2.4 Send Data#
# Send test messages
for i in range(10):
message = "K230 UDP client send test {0} \r\n".format(i)
print("Sending:", message)
# Encode the message into bytes and send
bytes_sent = s.sendto(message.encode(), addr)
print("Bytes sent:", bytes_sent)
# Pause for a while before the next send
time.sleep(0.2)
In the loop, the program generates test messages and sends them to the specified server address using the sendto
function. The message needs to be converted into a byte string before sending. After sending successfully, the number of bytes sent is printed, and a short delay is set.
2.5 Close Socket#
# Close the socket
s.close()
print("Client ended.")
After the data is sent, the socket is closed to release resources.
2.6 Complete Example#
import socket
import os
import time
import network
def network_use_wlan(is_wlan=True):
if is_wlan:
sta = network.WLAN(0)
sta.connect("Canaan", "Canaan314")
print(sta.status())
while sta.ifconfig()[0] == '0.0.0.0':
os.exitpoint()
print(sta.ifconfig())
ip = sta.ifconfig()[0]
return ip
else:
a = network.LAN()
if not a.active():
raise RuntimeError("LAN interface is not active.")
a.ifconfig("dhcp")
print(a.ifconfig())
ip = a.ifconfig()[0]
return ip
def udpclient():
# Configure network interface
network_use_wlan(True)
# Get server address and port number
ai = socket.getaddrinfo('192.168.1.110', 8080)
print("Address infos:", ai)
addr = ai[0][-1]
print("Connect address:", addr)
# Create UDP socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
for i in range(10):
message = "K230 UDP client send test {0} \r\n".format(i)
print("Sending:", message)
# Send string
bytes_sent = s.sendto(message.encode(), addr)
print("Bytes sent:", bytes_sent)
time.sleep(0.2)
# Close the socket
s.close()
print("Client ended.")
# Start the client
udpclient()
3. Run and Test#
Use NetAssist Network Debugging Assistant to establish a UDP connection:
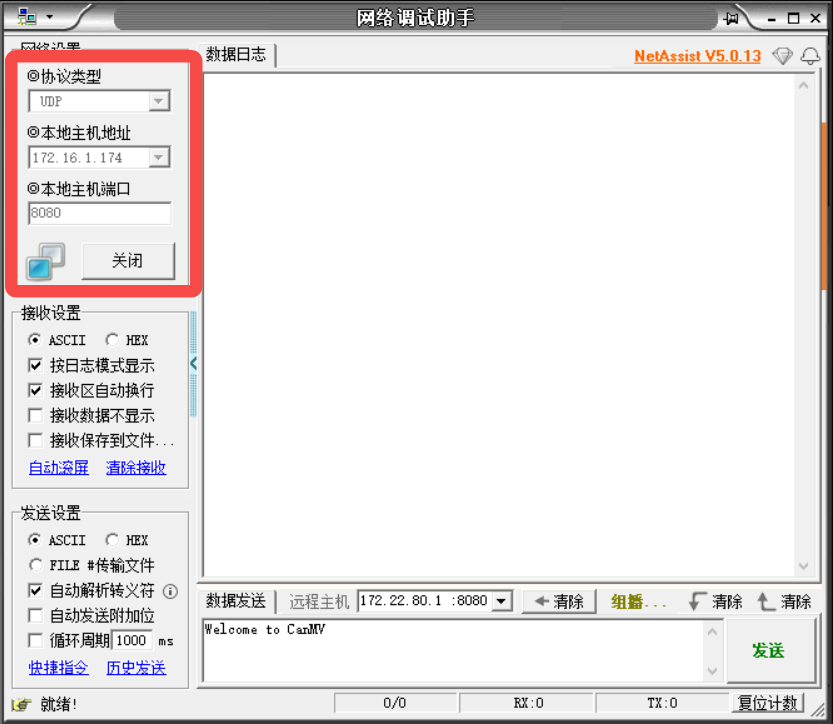
Modify the server IP address in the code:
ai = socket.getaddrinfo("172.16.1.174", 8080)
After running the example, NetAssist will display the received UDP packets:
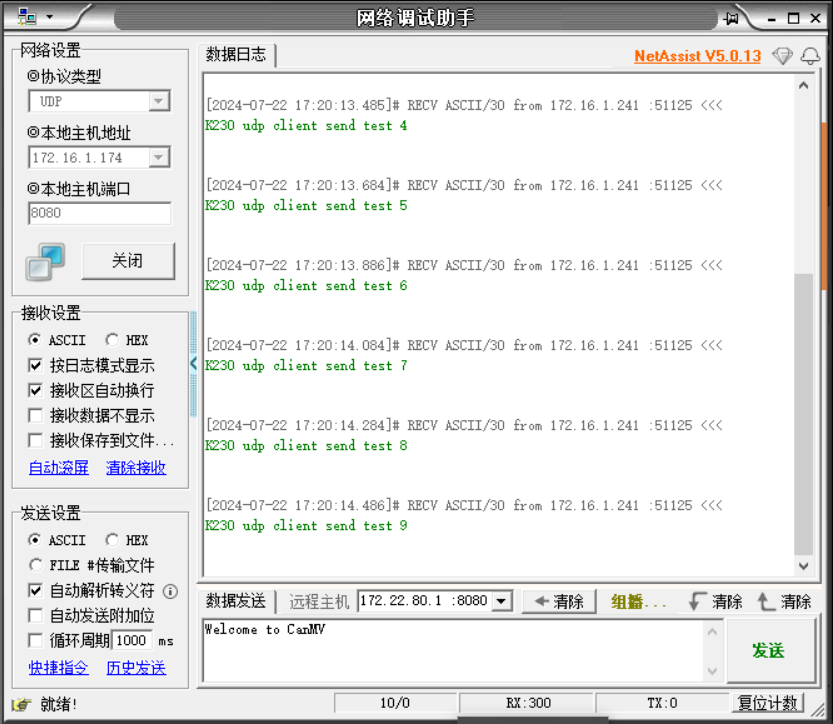